PHASE-2
In Phase 2 of my rover project, I equipped the rover with sensors
to enable autonomous functionalities, this included
ultrasonic sound sensor and LDRs.
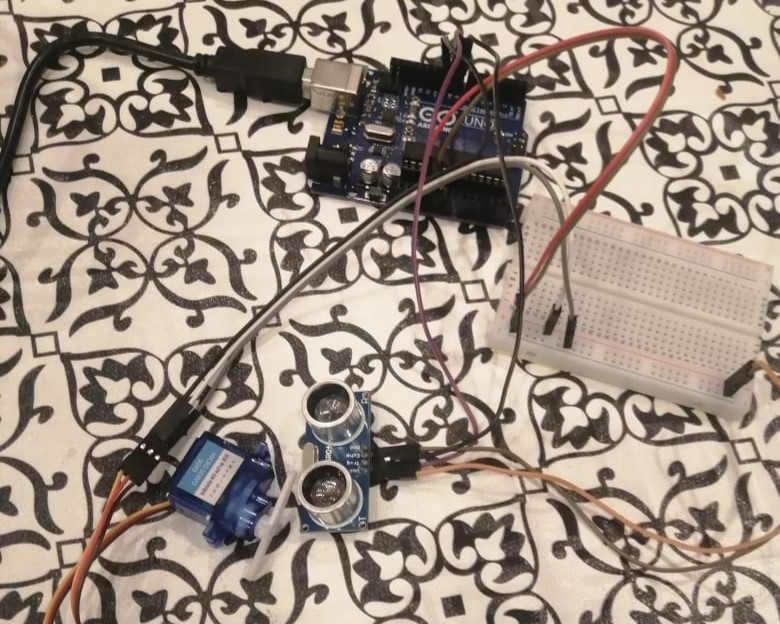
Ultrasonic Sound Sensor
The ultrasonic sound sensor plays a pivotal role in advancing the autonomy of the Rover. By linking the sensor to the servos, the system triggers servo movement when the distance falls below a specified threshold, enabling dynamic and responsive behavior in navigating obstacles autonomously.
In this version:
- The code checks the distance measured by the ultrasonic sensor.
- If the distance is greater than or equal to 80 cm (distance >= 80), it increments the servo positions (pos) by 15 degrees (pos = pos + 15).
- If the distance is less than or equal to 75 cm (distance <= 75), it decrements the servo positions (pos) by 15 degrees (pos = pos - 15).
- Servo positions are then updated (s1.write(pos), s2.write(pos), s3.write(pos), s4.write(pos)) accordingly to reflect the new position.
This code snippet illustrates how the ultrasonic sensor interacts with the servos to control the prototype's movements based on detected distances, thereby enhancing its autonomous navigation capabilities.
Max Distance Algorithm
I have integrated the ultrasonic sensor with the servo motor, implementing an algorithm designed to detect distances falling below a specified threshold. When triggered, the servo sequentially moves from 90 degrees to 0, returns to 90 degrees, proceeds to 180 degrees, and returns once more to 90 degrees. Throughout this movement sequence, the sensor actively scans to identify the maximum distance encountered. This critical data is then recorded and stored for further analysis and autonomous navigation decisions.
- Variables Initialization:
- Loop Function (loop()):
- Moves to 90 degrees (moveToPosition(90)).
- Moves to 0 degrees (moveToPosition(0)).
- Returns to 90 degrees (moveToPosition(90)).
- Moves to 180 degrees (moveToPosition(180)).
- Finding the Longest Distance:
- After completing the sequence of movements, the code retrieves the distance from the sensor again (distanceAfterMoves).
- It then compares
distanceAfterMoves
withlongestDistance
. - If
distanceAfterMoves
is greater thanlongestDistance
, it updateslongestDistance
todistanceAfterMoves
and records the corresponding servo position (posSensor) inlongestPosition
. - Servo Control Functions:
moveToPosition(int position)
: This function is placeholder for actual servo control code to move the servo to the specified position.- Distance Sensing Function:
getDistance()
: Placeholder function to read and return the current distance from the sensor.
longestDistance
: Stores the longest distance detected during the sequence of servo movements.
longestPosition
: Stores the servo position (posSensor) corresponding to the longestDistance.
-
1. The loop continuously reads the current distance from the sensor using
getDistance()
2. If the current distance (currentDistance) is less than 100, indicating an obstacle within range, the servo executes a predefined sequence of movements: